Wednesday, November 2, 2016
Remote Control Circuit Using Arduino
Remote Control Circuit Using Arduino
In this post, we are going to construct a remote control circuit for controlling home appliances using arduino microcontroller.
By: Girish Radhakrishnan
This circuit can turn on/off your gadgets using TV remotes unused buttons or any other unused remote that may be lying in your junk box for ages.
The motto of this project is to help physically challenged persons, and help them to access the ON/OFF switching of the basic home appliances such as fans or lights independently.
The second objective is to enable the user to control the gadgets Like a boss without having to move from his or her existing position.
The circuit utilizes traditional IR based communication between transmitter and receiver.
This circuit is cent percent fool proof to other IR remotes, and other IR sources and less susceptible to errors.
The major problem with non-microcontroller based IR remote control circuit, which is found around the internet, is that it could turn ON/OFF with any IR based remote and can only control one device at an instant and also more susceptible to errors.
This circuit overcomes above specified issues, and we can control several gadgets on one remote and assign keys for specific gadgets.
Before proceeding this project you need to download the library files for arduino form this link and follow the instruction given below: https://github.com/z3t0/Arduino-IRremote
Instructions:
1) Click clone or download button form the given link and hit Download ZIP.
2) Extract the file and move IRremote folder to your library folder of Arduino.
3) Delete RobotIRremote folder from your arduino library. RobotIRremote has similar definition of IRremote library which clash and not able to upload the code to Arduino so, deletion/removal is mandatory.
By duplicating the above instruction your Arduino IDE software is ready for any/most of the IR based projects.
Assign keys for remote:
In our TV remote each key has unique hexadecimal code, which is used to recognize which key is pressed for an operation. Before uploading the final code to Arduino, you need to find what the hexadecimal codes for your keys are.
To do this construct the following circuit in breadboard and follow the instruction.
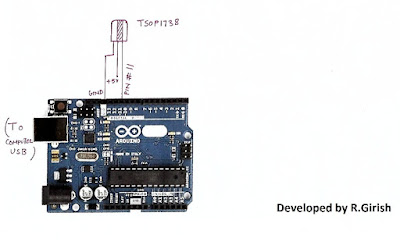
1) Open Arduino IDE and upload example code IRrecv Demo
2) Open serial monitor and press the key on remote that you want to use.
Youll see hexadecimal code pop up as soon as you press the key. Thats the hexadecimal code for that particular key.
3) Do the same for other two keys (3 keys are given in this project for controlling 3 devices)
· We are going to use these hexadecimal codes in the main program and upload to arduino.
//-----------------Program developed by R.Girish-----------//
#include<IRremote.h>
int input = 11;
int op1 = 8;
int op2 = 9;
int op3 = 10;
int intitial1;
int intitial2;
int intitial3;
IRrecv irrecv(input);
decode_results dec;
#define output1 0x111 // place your code received from button A
#define output2 0x112 // place your code received from button B
#define output3 0x113 // place your code received from button C
void setup()
{
irrecv.enableIRIn();
pinMode(op1,1);
pinMode(op2,1);
pinMode(op3,1);
}
void loop() {
if (irrecv.decode(&dec)) {
unsigned int value = dec.value;
switch(value) {
case output1:
if(intitial1 == 1) {
digitalWrite(op1, LOW);
intitial1 = 0;
} else {
digitalWrite(op1, HIGH);
intitial1 = 1;
}
break;
case output2:
if(intitial2 == 1) {
digitalWrite(op2, LOW);
intitial2 = 0;
} else {
digitalWrite(op2, HIGH);
intitial2 = 1;
}
break;
case output3:
if(intitial3 == 1) {
digitalWrite(op3, LOW);
intitial3 = 0;
} else {
digitalWrite(op3, HIGH);
intitial3 = 1;
}
break;
}
irrecv.resume();
}
}
//--------------Program developed by R.Girish-----------//
NOTE:
In the program:
#define output1 0x111 // place your code received from button A
#define output2 0x111 // place your code received from button B
#define output3 0x111 // place your code received from button C
· Place your 3 unique codes from your remote in this place of 111, 112, and 113 and upload the code. Hexadecimal codes will be from 0 to 9 and A to F, for example: 20156, 26FE789, FFFFFF.
· Place your code with 0x (zero x).
Circuit diagram:
· Pressing key trips the relay ON and by pressing again it will turn off the relay.
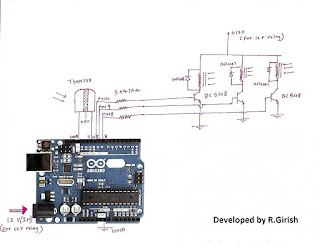
Available link for download